What is API?
API stands for application programming interface,
which is a set of definitions and protocols for building and integrating
application software. These interfaces establish a
secure and standardized means for different applications to collaborate, and exchange of information or functionality without requiring user intervention.
How does API work?
The working of an API can be explained
with a few simple steps:
- The client initiates the
requests via the APIs URI;
- Upon receiving the request,
the API initiates a call to the server;
- Subsequently, the server
responds by sending back the requested information to the API;
- API sends back the response
to client;

To clarify the concept with a
diagram,
consider a real-life analogy: think of an API as similar to a waiter in a restaurant. When you place an
order request, the API acts like the waiter, conveying your request to the
chef, retrieving the requested food items, and returning to you with the
fulfilled order. This
real-life scenario serves as a practical illustration of how an API facilitates
seamless communication and data exchange between different components.
Understanding Machine-to-Machine Authentication with OAuth
2 in EyeQuestion API
The EyeQuestion API requires
machine-to-machine authentication, using the OAuth 2 protocol. This means that
to access the API endpoints, the requesting machine must obtain a token to
identify itself.
To illustrate this see the diagram below:
In the flowchart above you see how the
authentication flow works with the following steps:
- Send
client secret + Id to authorization server;
- Server
sends back bearer token;
- You
can now add the bearer token to the request header when calling an endpoint;
- API
returns response.
This authentication flow ensures secure
communication between machines, allowing you to seamlessly integrate and
utilize EyeQuestion's API functionalities in your application.
A common resource with a vast amount of information regarding OAUTH
authentication can be found here.
HTTP Response Codes
When interacting with the EyeQuestion
API, all endpoints will return default HTTP response codes like 200, 201, 204,
400, 403, 405, 422 and 500.
Below you’ll find what each of them
means.
Field
|
|
200
|
Ok – The request was executed
successfully
|
201
|
Created –
The request was executed successfully
|
204
|
No Content - The request was
successful, but there is no content to send in the response.
|
400
|
Bad request
– A common cause is a problem in the data sent to the API. Such as a wrong URL parameter or incorrect JSON.
|
403
|
Forbidden – This indicates a
problem with the authorization.
|
405
|
Method not
allowed - Most common causes are the incorrect specification of the request
being a GET, POST or PUT
|
422
|
Unprocessable Entity – There is a problem in the data
sent to the server.
|
500
|
Internal
server error – This usually refers to an unexpected error.
|
How to use API in
EyeQuestion using Swagger
There are many ways to connect to the
EyeQuestion API (using any application that supports OAuth2 protocol) like
Swagger and Postman. For efficient interaction we have a Swagger documentation
page. To access this documentation, please contact our support team at
support@eyequestion.nl.
This tutorial will help you get started
with using the EyeQuestion API. Follow the steps below to seamlessly integrate
EyeQuestion's functionalities into your application.
Step 1: Obtain OAuth 2 CredentialsFirst, you need to get your OAuth 2
credentials, for using API from EyeQuestion. This includes the client ID and
client secret. To do this, please contact us at support@eyequestion.nl.
Step 2: Retrieve Bearer Token
Next, you need to retrieve a bearer token:- Go to the Authentication Controller;
- Find the call to "Retrieve bearer token" and click on
"Try it out";
- Enter the client ID and client secret obtained in Step 1;
- Press "Execute" to retrieve the bearer token.
Step 3: Authorize- Copy the bearer token received in Step 2 from the response
body;
- Click on "Authorize";
- Paste the token in this format: Bearer {token}. (See image
below);
- Press "Authorize";
- Click “Close”.
Step 4: Make Your First API Call
You are now authorized and can start making
API calls.
For example, let's retrieve the list of all
projects using the "Get projects" API endpoint.
This call retrieves list of projects based
on status, field value and change date.
The “changedSince” and “changedBefore” dates don’t just check when the most
recent edit to the project has taken place, it also takes into account when the
most recent data was collected.
In this API call, all parameters are
optional. Here's are the steps to follow:- Go to the Project Controller;
- Click on “Get projects”;
- Click on "Try it out";
- Press "Execute";
- All projects matching the criteria will be returned.
To
understand the meaning of each key, click on "Model". This will
display a detailed description of what each key represents.

How to import endpoints in Postman (or another
application)
To enhance usability, these API endpoints
can be easily imported into your preferred application. This example
demonstrates how to import these endpoints into Postman.
Here are the steps to
follow:
- Click on the link shown in the
image below. This link will direct you to the JSON data containing all the
endpoints.
- Copy the JSON data and save it
into a .txt file;
- Go to Postman, click on
“Import”;
- Choose the file to import;
- All the endpoints will be
imported in Postman.
Postman
The EyeQuestion API endpoints are now ready to
be used in Postman.
Next,
- Go to EyeQuestion
API
- Go to "Variables
- Add the variable
{baseUrl} and provide both the initial and current values
- Click on “Save”
to apply the changes
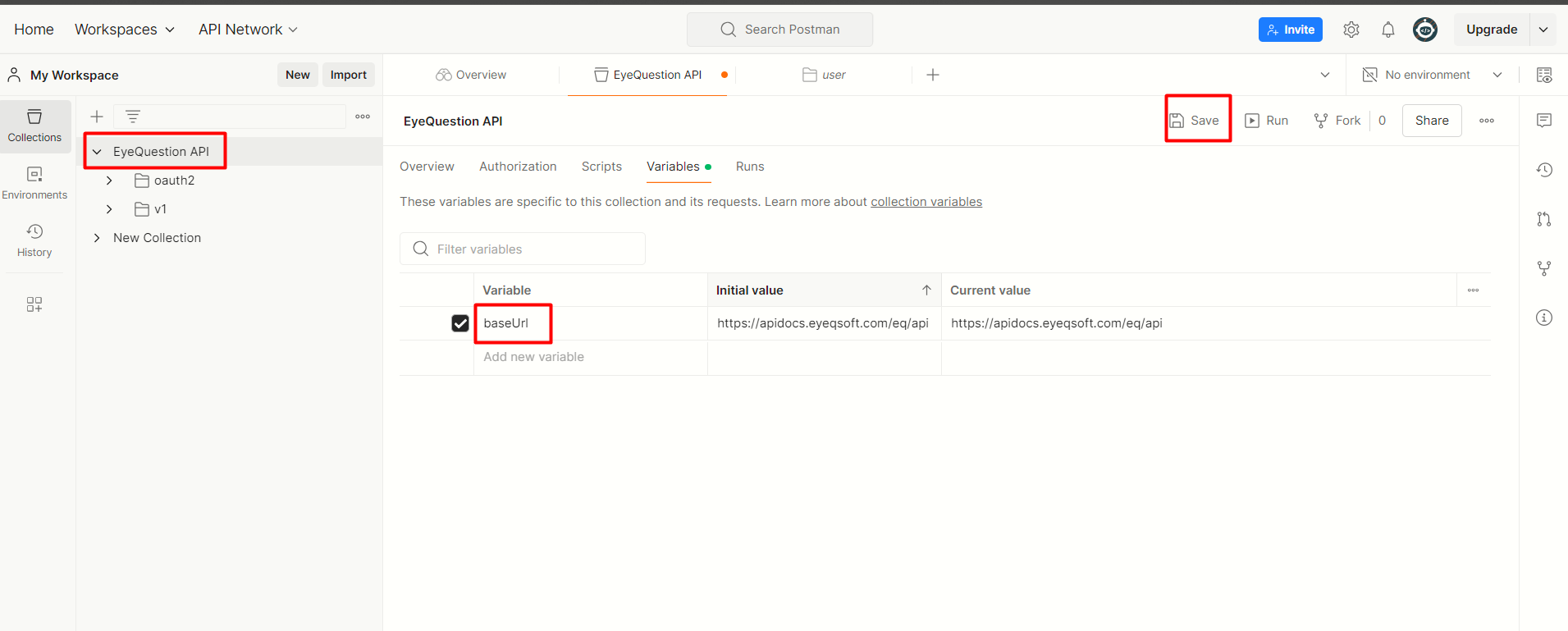
After setting up the baseUrl,
- Go to the end point “Retrieve bearer token”
- Enter the client_id and client_secret
- Click “Send"
- Response will contain the token
- Copy the token and go to EyeQuestion API (as shown
in the second image below)
- Go to “Variables”
- Add a new variable named “apiKey” with value
Bearer {token}
- Click “Save” to apply the changes


To make the first API call, lets retrieve the projects,- Go to “Get projects” endpoint
- If you want to retrieve all projects without any
filters, uncheck the "Query Params" option
- Click
“Send"
- The response will return a list of all projects